03-Quadratics
In this article we will look at the (simple) quadratics included in the RenderMan Interface. These for the time being will be our default primitives when used in various scenes, at least until we get to more complex surfaces.
Blocking
The RenderMan Interface is quite large so I’ll only point out interesting bits of it as we go. The following go program creates a frame per (simple) quadratic type, sphere, disk, torus, cylinder and cone. The program makes use of the FrameBegin / FrameEnd pair to define seperate frames each containing their own world data.
FrameBegin frame – where frame is a sequence number, usually per scene. Use this block to place frame specific commands before the WorldBegin / WorldEnd pair. For instance you probably want to set the output filename per frame.
RIB generation of individual quadratic objects
/* renderman/fromscratch/quadratics.go
* mae 012018
*/
package main
import (
"flag"
"fmt"
"os"
"strings"
"reflect"
"gitlab.com/mae.earth/renderman/fromscratch/library/format"
)
const Version string = "renderman/fromscratch/quadratics program 1"
func main() {
scale := flag.Float64("scale", 1.0, "`scale of output image")
maxsamples := flag.Int("maxsamples", 512, "maxsamples for raytrace hider")
minsamples := flag.Int("minsamples", 16, "minsamples for raytrace hider")
red := …
In this program I have wrapped up the parameterlist of each command into a seperate function to be formatted based on type. RIB and specifically the RIB parsers can make use of a compact floating point notation. This makes hand-editing much easier but also I feel gives a professional look to any generated RIB scene.
At this point I’ll introduce the seperate code library we will be using to create our toolkit. The first use is to format float64 into this compact RIB notation.
import "gitlab.com/mae.earth/renderman/fromscratch/library/format"
fmt.Printf("1.23 golang=%f, RIB=%s\n",1.23,format.Float64(1.23))
fmt.Printf("0.45 golang=%f, RIB=%s\n",0.45,format.Float64(0.45))
fmt.Printf("-0.1 golang=%f, RIB=%s\n",-0.1,format.Float64(-0.1))
-- output
1.23 golang=1.230000, RIB=1.23
0.45 golang=0.450000, RIB=.45
-0.1 golang=-0.10000, RIB=-.1
##RenderMan
##Machine Generated By "quadrics.go"
##Scene quadrics.rib
version 3.04
Display "beginning.exr" "openexr" "rgba"
Format 200 200 1
Projection "perspective" "float fov" [60]
Hider "raytrace" "int maxsamples" [512] "int minsamples" [16] "int incremental" [100]
Integrator "PxrPathTracer" "example"
PixelFilter "gaussian" 4 4
ArchiveBegin "lighting"
AttributeBegin
Attribute "identifier" "name" "fill_light"
Translate 0 15 0
Scale 3 3 3
Light "PxrSphereLight" "fill_light" "float intensity" [8] "float temperature" [7500]
AttributeEnd
AttributeBegin
Attribute "identifier" "name" "key_light"
Translate 0 0 -5
Scale 3 3 3
Light "PxrSphereLight" "key_light" "float intensity" [8] "float temperature" [3000]
AttributeEnd
ArchiveEnd
FrameBegin 1
Display "sphere.exr" "openexr" "rgba"
WorldBegin
Translate 0 0 3.5
ReadArchive "lighting"
AttributeBegin
Attribute "identifier" "name" "sphere"
Bxdf "PxrDiffuse" "surface" "color diffuseColor" [1 0 0]
Sphere 1 -1 1 360
AttributeEnd
WorldEnd
FrameEnd
FrameBegin 2
Display "cone.exr" "openexr" "rgba"
WorldBegin
Translate 0 0 3.5
ReadArchive "lighting"
AttributeBegin
Attribute "identifier" "name" "cone"
Bxdf "PxrDiffuse" "surface" "color diffuseColor" [1 0 0]
Cone 3 1 360
AttributeEnd
WorldEnd
FrameEnd
FrameBegin 3
Display "cylinder.exr" "openexr" "rgba"
WorldBegin
Translate 0 0 3.5
ReadArchive "lighting"
AttributeBegin
Attribute "identifier" "name" "cylinder"
Bxdf "PxrDiffuse" "surface" "color diffuseColor" [1 0 0]
Cylinder 1 -1 1 360
AttributeEnd
WorldEnd
FrameEnd
FrameBegin 4
Display "disk.exr" "openexr" "rgba"
WorldBegin
Translate 0 0 3.5
ReadArchive "lighting"
AttributeBegin
Attribute "identifier" "name" "disk"
Bxdf "PxrDiffuse" "surface" "color diffuseColor" [1 0 0]
Disk 1 1 360
AttributeEnd
WorldEnd
FrameEnd
FrameBegin 5
Display "torus.exr" "openexr" "rgba"
WorldBegin
Translate 0 0 3.5
ReadArchive "lighting"
AttributeBegin
Attribute "identifier" "name" "torus"
Bxdf "PxrDiffuse" "surface" "color diffuseColor" [1 0 0]
Torus 1 6 0 360 360
AttributeEnd
WorldEnd
FrameEnd
We will in the future use more useful visualisations for the statements in a particular RIB scene :-
Blocking Example
- RenderMan RIB
- Preample
-
- Archive "lighting"
-
- Attribute "fill_light"
- Attribute "key_light"
-
- Frame 1 — "sphere.exr"
-
- World
-
- ReadArchive "lighting"
- Attribute "sphere"
-
- Frame 2 — "cone.exr"
-
- World
-
- ReadArchive "lighting"
- Attribute "cone"
-
- Frame 3 — "cylinder.exr"
-
- World
-
- ReadArchive "lighting"
- Attribute "cylinder"
-
- Frame 4 — "disk.exr"
-
- World
-
- ReadArchive "lighting"
- Attribute "disk"
-
- Frame 5 — "torus.exr"
-
- World
-
- ReadArchive "lighting"
- Attribute "torus"
I call this process (of visualisation) statement blocking or blocking statements; for the moment we can do this by hand, but a tool will be required for larger RIB scenes. However that naturally has to wait until we have a RIB parser in our toolbox.
Quadratics
Sphere
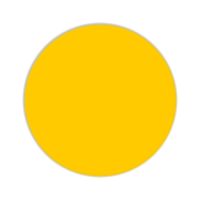
Sphere
Sphere 1 -1 1 360 RiSphere(float radius, float zmin, float zmax, float tmax, ...)
Exploring the parameters of Sphere
So last time we employed the humble sphere in our example RIB scene; the sphere surface in itself is very capable of producing a wide range of shapes. The following program generates a gallery view of some of the tweaked parameters.
RIB generation of various sphere parameters
/* renderman/fromscratch/quadratics_params.go
* mae 012018
*/
package main
import (
"flag"
"fmt"
"os"
"strings"
"reflect" …
This program generates shapes from the sphere RIB command, but can be readily altered for the rest of the quadratics to explore their parameters.
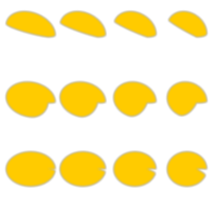
Params
Cone
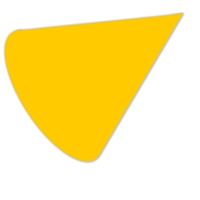
Cone
Cone 1 1 360 RiCone(float height, float radius, float tmax, ...)
One thing to note about the cone is that the base is non existant. However, the disk (described later) can be used to construct an apparent solid cone.
Torus
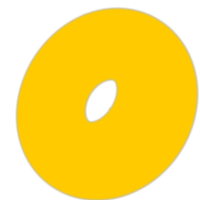
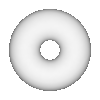
Torus w/animation
Torus 1 .6 0 360 360 RiTorus(float majrad, float minrad, float phimin, float phimax, float tmax, ...)
Cylinder
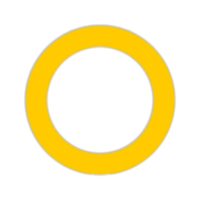
Cylinder
Cylinder 1 -1 1 360 RiCylinder(float radius, float zmin, float zmax, float tmax, ...)
As with the cone, the cylinder command does not include end-caps, but again the disk (or indeed the sphere) can be used to solve this problem.
Disk
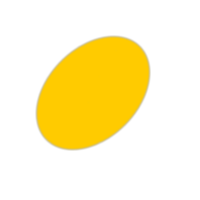
Disk
Disk 1 1 360 RiDisk(float height, float radius, float tmax, ...)
Animation
Making use of the introduced FrameBegin / FrameEnd pair we can produce a simple proceedural animation (see spinning torus above) as a sequence of frames.
RIB generation for simple animation
/* renderman/fromscratch/quadratics_ani.go
* mae 012018
*/
package main
import (
"flag"
"fmt"
"os"
"strings"
"reflect"
"gitlab.com/mae.earth/renderman/fromscratch/library/format"
)
const Version string = "renderman/fromscratch/quadratics_ani program 1"
func main() {
scale := flag.Float64("scale", 1.0, "`scale of output image")
maxsamples := flag.Int("maxsamples", 512, "maxsamples for raytrace hider")
minsamples := flag.Int("minsamples", 16, "minsamples for raytrace hider")
shape …
Some Ideas for the Reader
Using the animation program above do something more interesting. Play around with the parameters of the each quadratic type. Build larger scenes composed of many objects.
Next Time
We take a look at virtual cameras and the various RenderMan plugins.